Data Grid for .NET MAUI – Version 1.0.0 Demo
Published:
Modified:
NBA fan? This demo uses Monsalma DataGrid
control to display all NBA MVPs.
The source code is available on GitHub – https://github.com/Monsalma/Monsalma.Maui.Controls/tree/main/Monsalma-Maui-v010000.
To get started, please download Monsalma.Maui.Controls NuGet package: https://www.nuget.org/packages/Monsalma.Maui.Controls/1.0.0.
Additional resources:
Model (Data)
Instances of the Person
class (Monsalma_Maui_v010000.Data.Person
) contain the data:
public class Person
{
public int Row { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
}
View Model
MainViewModel
(Monsalma_Maui_v010000.ViewModels.MainViewModel
) randomly selects 100 NBA legends:
public MainViewModel()
{
Random random = new(DateTime.Now.Millisecond);
Persons = new();
int maxRandomNumber = Math.Min(FIRST_NAMES.Count, LAST_NAMES.Count);
for (int i = 0; i < 100; ++i)
{
int rnd = random.Next(maxRandomNumber);
Person p = new()
{
Row = i + 1,
FirstName = FIRST_NAMES[rnd],
LastName = LAST_NAMES[rnd]
};
Persons.Add(p);
}
}
View (XAML)
MainPage
(Monsalma_Maui_v010000.MainPage
) is what we’re interested in.
First we import the package (Monsalma.Maui.Controls
) and view model:
xmlns:controls="clr-namespace:Monsalma.Maui.Controls;assembly=Monsalma.Maui.Controls"
xmlns:viewmodels="clr-namespace:Monsalma_Maui_v010000.ViewModels"
Then we define row styles (header row, odd row, even row and selected row) for the data grid control:
<ContentPage.Resources>
<Style
TargetType="controls:DataGridRowHeader"
x:Key="HeaderRowStyle">
<Setter
Property="CellBackgroundColor"
Value="{StaticResource Key=Primary}" />
<Setter
Property="CellForegroundColor"
Value="{StaticResource Key=White}" />
</Style>
<Style
TargetType="controls:DataGridRowRegular"
x:Key="RegularOddRowStyle">
<Setter
Property="CellBackgroundColor"
Value="{StaticResource Key=White}" />
<Setter
Property="CellForegroundColor"
Value="{StaticResource Key=Black}" />
</Style>
<Style
TargetType="controls:DataGridRowRegular"
x:Key="RegularEvenRowStyle">
<Setter
Property="CellBackgroundColor"
Value="{StaticResource Key=Gray100}" />
<Setter
Property="CellForegroundColor"
Value="{StaticResource Key=Black}" />
</Style>
<Style
TargetType="controls:DataGridRow"
x:Key="SelectedRowStyle">
<Setter
Property="CellBackgroundColor"
Value="{StaticResource Key=Yellow100Accent}" />
<Setter
Property="CellForegroundColor"
Value="{StaticResource Key=Black}" />
</Style>
</ContentPage.Resources>
After that, we add the data grid markup:
<controls:DataGrid
Items="{Binding Persons}"
SelectionMode="Multiple"
SelectedItem="{Binding SelectedPerson, Mode=TwoWay}"
SelectedItems="{Binding SelectedPersons, Mode=TwoWay}"
HeaderRowStyle="{StaticResource Key=HeaderRowStyle}"
RegularOddRowStyle="{StaticResource Key=RegularOddRowStyle}"
RegularEvenRowStyle="{StaticResource Key=RegularEvenRowStyle}"
SelectedRowStyle="{StaticResource Key=SelectedRowStyle}">
Lastly, we add the columns. For this demo, we’re using only the simplest column type (DataGridColumnText
), which under the hub contains a Label
instance:
<controls:DataGrid.Columns>
<controls:DataGridColumnText
Width="Auto"
MinWidth="100"
MaxWidth="200"
DataBinding="Row"
HeaderText="#" />
<controls:DataGridColumnText
Width="*"
MinWidth="100"
DataBinding="FirstName"
HeaderText="First Name" />
<controls:DataGridColumnText
Width="*"
MinWidth="100"
DataBinding="LastName"
HeaderText="Last Name" />
</controls:DataGrid.Columns>
Things to note at this point:
- Column width is defined using
Width
,MinWidth
andMaxWidth
bindable properties.
For example,Row
column will take as much space as it needs (Width="Auto"
), but it will take at least 100 DIU (MinWidth="100"
) and will not take more than 200 DIU (MaxWidth="200"
).
More info at: https://monsalma.net/monsalma-controls-for-net-maui/monsalma-data-grid-for-net-maui-column-width/. Items
object acts as a data source for the grid (Items="{Binding Persons}"
). To specify the column data source, we useDataBinding
bindable property (DataBinding="FirstName"
).- We specify column header text with
HeaderText
(HeaderText="First Name"
).
Screenshots
Windows
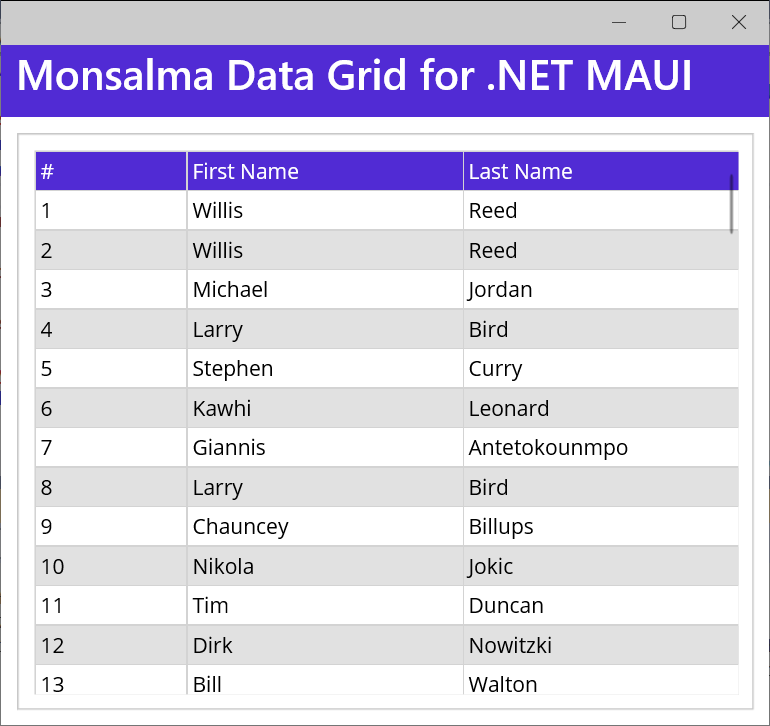
Android
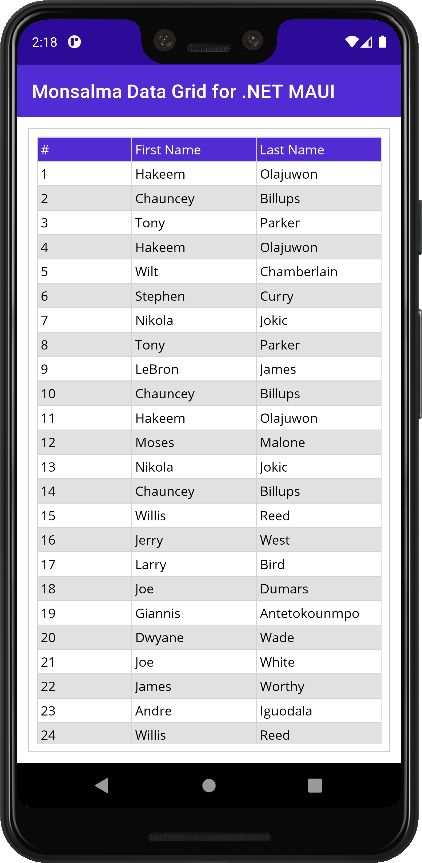
Leave a Reply